Commit fc589cb
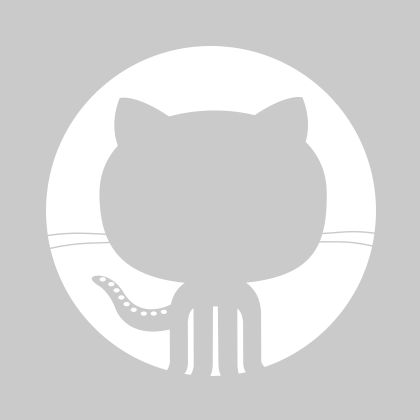
Gusted
1 parent d9f4383 commit fc589cb
6 files changed
+108
-5
lines changed+1
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
39 | 39 |
| |
40 | 40 |
| |
41 | 41 |
| |
| 42 | + | |
42 | 43 |
| |
43 | 44 |
| |
44 | 45 |
| |
|
+47
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
13 | 13 |
| |
14 | 14 |
| |
15 | 15 |
| |
| 16 | + | |
16 | 17 |
| |
17 | 18 |
| |
18 | 19 |
| |
19 | 20 |
| |
| 21 | + | |
| 22 | + | |
20 | 23 |
| |
21 | 24 |
| |
22 | 25 |
| |
| |||
225 | 228 |
| |
226 | 229 |
| |
227 | 230 |
| |
| 231 | + | |
| 232 | + | |
| 233 | + | |
| 234 | + | |
| 235 | + | |
| 236 | + | |
| 237 | + | |
| 238 | + | |
| 239 | + | |
| 240 | + | |
| 241 | + | |
| 242 | + | |
| 243 | + | |
| 244 | + | |
| 245 | + | |
| 246 | + | |
| 247 | + | |
| 248 | + | |
| 249 | + | |
| 250 | + | |
| 251 | + | |
| 252 | + | |
| 253 | + | |
| 254 | + | |
| 255 | + | |
| 256 | + | |
| 257 | + | |
| 258 | + | |
| 259 | + | |
| 260 | + | |
| 261 | + | |
| 262 | + | |
| 263 | + | |
| 264 | + | |
| 265 | + | |
| 266 | + | |
| 267 | + | |
| 268 | + | |
228 | 269 |
| |
229 | 270 |
| |
230 | 271 |
| |
| |||
290 | 331 |
| |
291 | 332 |
| |
292 | 333 |
| |
| 334 | + | |
| 335 | + | |
| 336 | + | |
| 337 | + | |
| 338 | + | |
| 339 | + | |
293 | 340 |
| |
294 | 341 |
| |
295 | 342 |
| |
|
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
1328 | 1328 |
| |
1329 | 1329 |
| |
1330 | 1330 |
| |
| 1331 | + | |
| 1332 | + | |
| 1333 | + | |
| 1334 | + | |
| 1335 | + | |
| 1336 | + | |
| 1337 | + | |
| 1338 | + | |
| 1339 | + | |
| 1340 | + | |
| 1341 | + | |
| 1342 | + | |
| 1343 | + | |
| 1344 | + | |
| 1345 | + | |
| 1346 | + | |
| 1347 | + | |
| 1348 | + | |
| 1349 | + | |
| 1350 | + | |
| 1351 | + | |
| 1352 | + | |
| 1353 | + | |
| 1354 | + | |
| 1355 | + | |
| 1356 | + | |
| 1357 | + | |
| 1358 | + | |
| 1359 | + | |
| 1360 | + | |
| 1361 | + | |
| 1362 | + | |
| 1363 | + | |
| 1364 | + | |
| 1365 | + | |
| 1366 | + | |
| 1367 | + | |
| 1368 | + | |
| 1369 | + | |
| 1370 | + | |
| 1371 | + | |
| 1372 | + | |
| 1373 | + | |
| 1374 | + | |
| 1375 | + | |
| 1376 | + | |
| 1377 | + | |
| 1378 | + | |
| 1379 | + | |
| 1380 | + | |
| 1381 | + |
+5-5
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
165 | 165 |
| |
166 | 166 |
| |
167 | 167 |
| |
| 168 | + | |
168 | 169 |
| |
169 |
| - | |
170 | 170 |
| |
171 | 171 |
| |
172 | 172 |
| |
173 | 173 |
| |
| 174 | + | |
174 | 175 |
| |
175 |
| - | |
176 | 176 |
| |
177 | 177 |
| |
178 | 178 |
| |
| |||
3181 | 3181 |
| |
3182 | 3182 |
| |
3183 | 3183 |
| |
3184 |
| - | |
| 3184 | + | |
3185 | 3185 |
| |
3186 | 3186 |
| |
3187 | 3187 |
| |
3188 | 3188 |
| |
3189 | 3189 |
| |
3190 | 3190 |
| |
3191 |
| - | |
| 3191 | + | |
3192 | 3192 |
| |
3193 | 3193 |
| |
3194 | 3194 |
| |
| |||
3198 | 3198 |
| |
3199 | 3199 |
| |
3200 | 3200 |
| |
3201 |
| - | |
| 3201 | + | |
3202 | 3202 |
| |
3203 | 3203 |
| |
3204 | 3204 |
| |
|
+2
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
1 | 1 |
| |
2 | 2 |
| |
3 | 3 |
| |
| 4 | + | |
| 5 | + |
+2
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
| 1 | + | |
| 2 | + |
0 commit comments